Hello Bzot,
Generally speaking, the device has his base configuration.
You can modify it changing parameters
Speaking of Scan2Deploy, consider that you could also apply a configuration by exporting the content of a configuration as encrypted text and apply it to the device by sending an intent via Code or ADB:
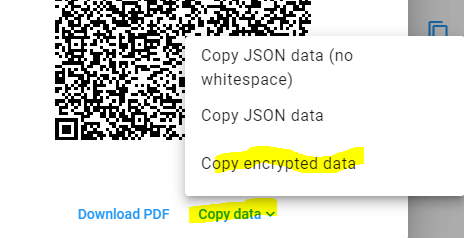
…
Intent i = new Intent("datalogic.scan2deploy.intent.action.START_SERVICE")
.putExtra("encoding", "v2")
.putExtra("data", "Bm9uiYc//NWOn...");
.setClassName("com.datalogic.scan2deploy", "com.datalogic.scan2deploy.S2dServiceReceiver");
getApplicationContext().sendBroadcast(i);
or:
adb shell am broadcast -a datalogic.scan2deploy.intent.action.START_SERVICE -n "com.datalogic.scan2deploy/.S2dServiceReceiver" --es encoding v2 --es data '...'
For more info, see:
NOTE:
Once configured via Scan2Deploy or SDK, to date there is no way to verify on the device GUI which “app profile” is currently applied.
The advantage of the Scan2Deploy Studio’s Application policies is that they allow to define one or more “per-app configuration profiles” that are automatically activated by the system when a specific application is active. They are usefull when you have multiple application that requires different device base setting.
In this way applications will no longer have to worry about “adapting the system configuration to their operation” (often requiring the use of the SDK), because with the appropriate Application policies defined at the system level, it will be the system that will automatically reconfigure itself depending on the active application.
About the “good” / “bad” beeps, by default the system is set to notify any decoded label as a “goodread”, playing a beep and turning-on for a while the notification led and the GoodReed green light on the code label.
These behaviors are controlled by three different settings under Setting / Datalogic Setting / Scanner & decoder / GoodRead:
- Enable good read (which enables the “goodread” beep)
- Enable Green Spot
- Enable good read LED
Optionally, if available on the device, you can also activate the vibrator as a GoodRead notification.
The menus under Setting / Datalogic Setting / Scanner & decoder / Notification allows to finetune the built-in good read notification.
The same settings are available also in the SDK or via intents through the ConfigurationManager and the PropertyID classes. (see: GOOD_READ_ENABLE , GREEN_SPOT_ENABLE, etc…)
If you want to play different “good” / “bad” beeps based on some logic by you application, you have to disable the automatic good read audio feedback, validate the code received through the onRead event (or through the intent you configured in the IntentWedge) and then playing the sound you prefer by using the Android Standard APIs.
Finally, regarding the last question, yes, it is sufficient.
If there are no other active applications in the system that use the SDK to receive barcodes (or if they are closed or in the background and have themselves deregistered their listeners), deselecting all listeners in the Wedge menu (disable Keyboard Wedge, Intent Wedge, and Web Wedge) is sufficient to disable the scanner.
Simone
L3 - Mobile Products Specialist SW Engineer